How to Use the L293D Motor Shield Driver with Arduino
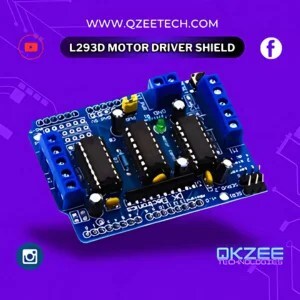
What is L293D Motor Driver Shield
Understanding How the L293D Motor Driver Shield Works
If you’re planning to build a robot, you’ll need to control various motors like DC motors, stepper motors, or servos, and the L293D Motor Driver Shield is the best option for this task. This shield is not only capable of handling these motor types but also eliminates the need for additional modules. The brains of this shield are its two L293D motor drivers and a 74HC595 shift register, which work together seamlessly. I’ve found this combination particularly useful for automating motorized systems in my own projects.
The L293D is a dual-channel H-Bridge motor driver, meaning it can control up to four DC motors or two stepper motors. The 74HC595 shift register, on the other hand, extends the Arduino’s digital pins by converting its four pins into eight direction control pins, making it easy to manage multiple motors. With these features, the L293D Shield ensures precision and efficiency for both simple and complex robotic designs.
How to Use L293D Motor Driver with Arduino
The L293D Motor Driver Shield is a versatile and popular component designed for controlling various types of motors in Arduino projects. It simplifies motor operations, especially when dealing with DC motors, by acting as a reliable interface between the Arduino and the motors. When I first started using it, I appreciated how its compact design made it easy to connect and manage multiple motors without needing complex circuits or external power regulation.
This tutorial covers how to control a DC motor using the L293D Motor Driver Shield and Arduino. To set it up, you simply mount the shield onto your Arduino, connect your motor wires to the output pins, and upload a control code. The L293D handles the power and direction control efficiently, making it ideal for projects like robots, conveyor belts, or any automated system requiring precise motor control.
Components for Using L293D Motor Driver with Arduino UNO
Essential Parts for Motor Control Projects
- L293D Motor Driver Shield: The main component to control motor functions seamlessly.
- DC Motor: Provides the motion, essential for motorized projects.
- I2C LCD Display: Displays real-time feedback, helpful for debugging and monitoring.
- Push Buttons: Allows for interactive controls in your setup.
- POT (Potentiometer): Adjusts motor speed for customization.
- Breadboard: Organizes connections for a clean and manageable layout.
- Jumper Wires: Provides flexible and reliable connections between components.
Features of L293D Motor Driver
- Dual H-Bridge IC: Controls up to two DC motors or one stepper motor independently.
- Bidirectional Control: Allows motors to move in both directions for versatile movement.
- Current Handling: Each channel can handle up to 600mA of continuous current and 1.2A peak current.
- Voltage Range: Operates between 4.5V to 36V, providing flexibility for different motor types.
- Protection Diodes: Built-in diodes protect the IC from back EMF generated by motors.
- PWM Support: Allows for Pulse Width Modulation (PWM) to control motor speed precisely.
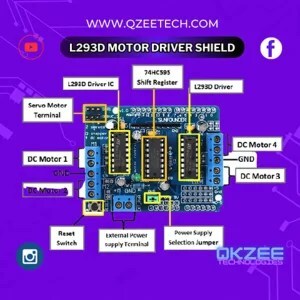
Motor Output Pins
- M1, M2, M3, M4: These pins control the rotation and direction of the motors connected to the L293D Motor Driver Shield.
Control Pins
- IN1, IN2, IN3, IN4: These pins connect to the Arduino and control the motor direction by setting them HIGH or LOW.
- ENA and ENB Pins: These pins enable motor channels, and applying a PWM signal helps control motor speed.
Power Supply Pins
- VCC: Provides power to the motors.
- GND: Connects to the ground of both the Arduino and external power supply.
Servo Motor Pins
- The shield provides three sets of pins for connecting servo motors, including 5V, GND, and signal connections.
I2C Pins
- SDA and SCL: These pins enable communication between the Arduino and other I2C-compatible devices for expanded functionality.
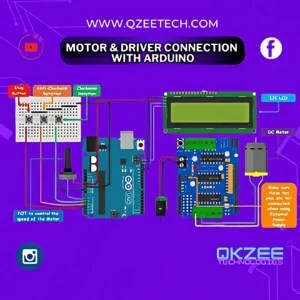
Controlling a DC Motor with Arduino UNO and L293D Motor Driver Shield
Connecting a DC Motor to an Arduino Uno using the L293D Motor Driver Shield opens up a world of possibilities for motor control. Whether you’re working with speed adjustments or directional changes, integrating components like an I2C LCD display, potentiometer (POT), and buttons adds precision and flexibility to your project. Below is a simplified guide to setting up this system for seamless operation.
Key Components and Setup
DC Motor and Motor Control:
Connect the DC Motor to the M3 motor output terminal of the L293D Motor Driver Shield. This configuration allows precise control of motor speed and rotation direction, including clockwise and anti-clockwise rotation, using digital input pins.Buttons for Control:
Use three buttons for motor commands:- Clockwise Rotation Button connected to a digital input pin on the Arduino.
- Anti-Clockwise Rotation Button linked to another digital pin.
- Stop Button for halting the motor operation.
Potentiometer (POT) for Speed Adjustment:
The potentiometer regulates speed by connecting one side to 5V, the other to GND, and the middle pin to an analog input pin (e.g., A0).I2C LCD Display:
For visual feedback, link the I2C LCD display to the Arduino via SDA and SCL pins, ensuring efficient communication.External Power Supply:
An external 5V power supply connects to the L293D Motor Driver Shield’s power terminals to ensure sufficient power for the motor. Remember to disconnect the jumper between the Arduino 5V and shield power when using an external source.Arduino and Shield Alignment:
Mount the L293D Motor Driver Shield on the Arduino Uno, ensuring all pins align. Power the Arduino via USB or another external power source.
By setting up these components with proper connections and leveraging the versatility of the L293D Motor Driver Shield, you can create a reliable and efficient motor control system tailored to your project’s needs.
Setting Up Arduino Code for Motor Control with the L293D Motor Driver Shield
#include
// Library to run DC Motor Using Motor Driver Shield
#include
// Library to Run I2C LCD
LiquidCrystal_I2C lcd(0x27, 16, 2); // Format -> (Address,Columns,Rows )
// Create the motor object connected to M3
AF_DCMotor motor(3);
// Define button pins
const int forwardButtonPin = A1;
const int reverseButtonPin = A2;
const int stopButtonPin = A3;
// Define potentiometer pin
const int potPin = A0;
// Variables to store motor state and direction
bool motorRunning = false;
int motorDirection = BACKWARD; // FORWARD or BACKWARD
// Read the potentiometer value
int potValue;
int motorSpeed;
// Variable to store button states
bool forwardButtonState;
bool stopButtonState;
bool reverseButtonState;
// Inline function to check if button is pressed packed with debouncing logic
inline bool chkButtonState(int pinNum, int checkState, int debounceDelay) {
if (((digitalRead(pinNum) == checkState) ? true : false) == true) {
delay(debounceDelay);
return (((digitalRead(pinNum) == checkState) ? (true) : (false)) == true);
} else {
return false;
}
}
void setup() {
// initialize the lcd
lcd.init();
// Turn on the Backlight
lcd.backlight();
// Clear the display buffer
lcd.clear();
// Set cursor (Column, Row)
lcd.setCursor(0, 0);
lcd.print("DC Motor using");
lcd.setCursor(0, 1);
lcd.print("L293D Shield");
// Set button pins as inputs
pinMode(forwardButtonPin, INPUT_PULLUP);
pinMode(stopButtonPin , INPUT_PULLUP);
pinMode(reverseButtonPin, INPUT_PULLUP);
// Start with motor off
motor.setSpeed(0);
motor.run(RELEASE);
delay(2000);
// Clear the display buffer
lcd.clear();
// Set cursor (Column, Row)
lcd.setCursor(0, 0);
lcd.print("Motor Direction:");
lcd.setCursor(0, 1);
lcd.print("Stopped ");
}
void loop() {
// Read the potentiometer value for changing speed as per Analog input
potValue = analogRead(potPin);
motorSpeed = map(potValue, 0, 1023, 0, 255);
// Read the button states
forwardButtonState = chkButtonState(forwardButtonPin, LOW, 20);
reverseButtonState = chkButtonState(reverseButtonPin, LOW, 20);
stopButtonState = chkButtonState(stopButtonPin, LOW, 20);
// check for Forward run
if (forwardButtonState && (!motorRunning || motorDirection == BACKWARD)) {
// Set cursor (Column, Row)
lcd.setCursor(0, 1);
lcd.print("Clock ");
if (motorDirection == BACKWARD) {
motor.setSpeed(0);
motor.run(RELEASE);
delay(1000);
}
motorRunning = true;
motorDirection = FORWARD;
motor.setSpeed(motorSpeed);
motor.run(FORWARD);
}
// check for Reverse run
else if (reverseButtonState && (!motorRunning || motorDirection == FORWARD)) {
// Set cursor (Column, Row)
lcd.setCursor(0, 1);
lcd.print("Anti-Clk");
if (motorDirection == FORWARD) {
motor.setSpeed(0);
motor.run(RELEASE);
delay(1000);
}
motorRunning = true;
motorDirection = BACKWARD;
motor.setSpeed(motorSpeed);
motor.run(BACKWARD);
}
// Stop motor
else if (stopButtonState && motorRunning) {
// Set cursor (Column, Row)
lcd.setCursor(0, 1);
lcd.print("Stopped ");
motorRunning = false;
motor.setSpeed(0);
motor.run(RELEASE);
}
// Adjust motor speed if running and display speed on LCD
if (motorRunning) {
motor.setSpeed(motorSpeed);
// Set cursor (Column, Row)
lcd.setCursor(9, 1);
lcd.print("SPD:");
lcd.print(((motorSpeed*100)/255));
lcd.print("% ");
}
}
Where to Buy Your I2C Module for LCD
Looking for affordable components for this Arduino project? Check out QKZee Technologies, an online shop in Lahore, Pakistan, offering the best components for students and DIY projects. Whether you’re looking for sensors, modules, or other electronics at a cheap price, they’ve got it all. Visit them at QKZeeTech.
What is the purpose of the L293D Motor Driver Shield in this setup?
The L293D Motor Driver Shield allows the Arduino Uno to control DC motors, including speed and rotation direction, using features like PWM (Pulse Width Modulation) and dual H-Bridge ICs.
How do I connect the DC Motor to the L293D Motor Driver Shield?
The DC Motor is connected to one of the motor output terminals, such as M3, on the shield. This enables bidirectional control and speed adjustments.
How is the motor speed controlled?
Motor speed is controlled using a potentiometer (POT) connected to an analog input pin on the Arduino (e.g., A0). Rotating the POT adjusts the voltage, which alters the speed.
Why do I need an external power supply?
An external 5V power supply provides sufficient power to the motor, especially when higher loads are required. Ensure the jumper connecting the Arduino's 5V and the shield’s power terminals is removed when using an external power source.
What are some applications of this motor control system?
This setup is ideal for robotics, automation projects, conveyor systems, and other applications requiring precise motor control and feedback.